mirror of
https://github.com/sockspls/badfish
synced 2025-05-01 09:13:08 +00:00
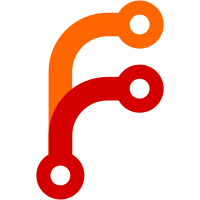
Previously, we had duplicated History: - one with (piece,to) called History - one with (from,to) called FromTo Now that we have only one, rename it to History, which is the generally accepted name in the chess programming litterature for this technique. Also correct some comments that had not been updated since the introduction of CMH. No functional change.
123 lines
3.9 KiB
C++
123 lines
3.9 KiB
C++
/*
|
|
Stockfish, a UCI chess playing engine derived from Glaurung 2.1
|
|
Copyright (C) 2004-2008 Tord Romstad (Glaurung author)
|
|
Copyright (C) 2008-2015 Marco Costalba, Joona Kiiski, Tord Romstad
|
|
Copyright (C) 2015-2016 Marco Costalba, Joona Kiiski, Gary Linscott, Tord Romstad
|
|
|
|
Stockfish is free software: you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation, either version 3 of the License, or
|
|
(at your option) any later version.
|
|
|
|
Stockfish is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#ifndef MOVEPICK_H_INCLUDED
|
|
#define MOVEPICK_H_INCLUDED
|
|
|
|
#include <algorithm> // For std::max
|
|
#include <cstring> // For std::memset
|
|
|
|
#include "movegen.h"
|
|
#include "position.h"
|
|
#include "types.h"
|
|
|
|
|
|
/// HistoryStats records how often quiet moves have been successful or unsuccessful
|
|
/// during the current search, and is used for reduction and move ordering decisions.
|
|
struct HistoryStats {
|
|
|
|
static const Value Max = Value(1 << 28);
|
|
|
|
Value get(Color c, Move m) const { return table[c][from_sq(m)][to_sq(m)]; }
|
|
void clear() { std::memset(table, 0, sizeof(table)); }
|
|
void update(Color c, Move m, Value v) {
|
|
|
|
if (abs(int(v)) >= 324)
|
|
return;
|
|
|
|
Square from = from_sq(m);
|
|
Square to = to_sq(m);
|
|
|
|
table[c][from][to] -= table[c][from][to] * abs(int(v)) / 324;
|
|
table[c][from][to] += int(v) * 32;
|
|
}
|
|
|
|
private:
|
|
Value table[COLOR_NB][SQUARE_NB][SQUARE_NB];
|
|
};
|
|
|
|
|
|
/// A template struct, used to generate MoveStats and CounterMoveHistoryStats:
|
|
/// MoveStats store the move that refute a previous one.
|
|
/// CounterMoveHistoryStats is like HistoryStats, but with two consecutive moves.
|
|
/// Entries are stored using only the moving piece and destination square, hence
|
|
/// two moves with different origin but same destination and piece will be
|
|
/// considered identical.
|
|
template<typename T, bool CM = false>
|
|
struct Stats {
|
|
const T* operator[](Piece pc) const { return table[pc]; }
|
|
T* operator[](Piece pc) { return table[pc]; }
|
|
void clear() { std::memset(table, 0, sizeof(table)); }
|
|
void update(Piece pc, Square to, Move m) { table[pc][to] = m; }
|
|
void update(Piece pc, Square to, Value v) {
|
|
|
|
if (abs(int(v)) >= 324)
|
|
return;
|
|
|
|
table[pc][to] -= table[pc][to] * abs(int(v)) / (CM ? 936 : 324);
|
|
table[pc][to] += int(v) * 32;
|
|
}
|
|
|
|
private:
|
|
T table[PIECE_NB][SQUARE_NB];
|
|
};
|
|
|
|
typedef Stats<Move> MoveStats;
|
|
typedef Stats<Value, true> CounterMoveStats;
|
|
typedef Stats<CounterMoveStats> CounterMoveHistoryStats;
|
|
|
|
|
|
/// MovePicker class is used to pick one pseudo legal move at a time from the
|
|
/// current position. The most important method is next_move(), which returns a
|
|
/// new pseudo legal move each time it is called, until there are no moves left,
|
|
/// when MOVE_NONE is returned. In order to improve the efficiency of the alpha
|
|
/// beta algorithm, MovePicker attempts to return the moves which are most likely
|
|
/// to get a cut-off first.
|
|
namespace Search { struct Stack; }
|
|
|
|
class MovePicker {
|
|
public:
|
|
MovePicker(const MovePicker&) = delete;
|
|
MovePicker& operator=(const MovePicker&) = delete;
|
|
|
|
MovePicker(const Position&, Move, Value);
|
|
MovePicker(const Position&, Move, Depth, Square);
|
|
MovePicker(const Position&, Move, Depth, Search::Stack*);
|
|
|
|
Move next_move();
|
|
|
|
private:
|
|
template<GenType> void score();
|
|
ExtMove* begin() { return cur; }
|
|
ExtMove* end() { return endMoves; }
|
|
|
|
const Position& pos;
|
|
const Search::Stack* ss;
|
|
Move countermove;
|
|
Depth depth;
|
|
Move ttMove;
|
|
Square recaptureSquare;
|
|
Value threshold;
|
|
int stage;
|
|
ExtMove *cur, *endMoves, *endBadCaptures;
|
|
ExtMove moves[MAX_MOVES];
|
|
};
|
|
|
|
#endif // #ifndef MOVEPICK_H_INCLUDED
|