mirror of
https://github.com/sockspls/badfish
synced 2025-05-06 19:39:34 +00:00
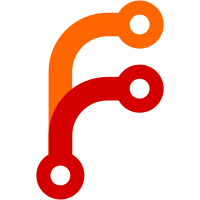
The new network caused some issues initially due to the very narrow neuron set between the first two FC layers. Necessary changes were hacked together to make it work. This patch is a mature approach to make the affine transform code faster, more readable, and easier to maintain should the layer sizes change again. The following changes were made: * ClippedReLU always produces a multiple of 32 outputs. This is about as good of a solution for AffineTransform's SIMD requirements as it can get without a bigger rewrite. * All self-contained simd helpers are moved to a separate file (simd.h). Inline asm is utilized to work around GCC's issues with code generation and register assignment. See https://gcc.gnu.org/bugzilla/show_bug.cgi?id=101693, https://godbolt.org/z/da76fY1n7 * AffineTransform has 2 specializations. While it's more lines of code due to the boilerplate, the logic in both is significantly reduced, as these two are impossible to nicely combine into one. 1) The first specialization is for cases when there's >=128 inputs. It uses a different approach to perform the affine transform and can make full use of AVX512 without any edge cases. Furthermore, it has higher theoretical throughput because less loads are needed in the hot path, requiring only a fixed amount of instructions for horizontal additions at the end, which are amortized by the large number of inputs. 2) The second specialization is made to handle smaller layers where performance is still necessary but edge cases need to be handled. AVX512 implementation for this was ommited by mistake, a remnant from the temporary implementation for the new... This could be easily reintroduced if needed. A slightly more detailed description of both implementations is in the code. Overall it should be a minor speedup, as shown on fishtest: passed STC: LLR: 2.96 (-2.94,2.94) <-0.50,2.50> Total: 51520 W: 4074 L: 3888 D: 43558 Ptnml(0-2): 111, 3136, 19097, 3288, 128 and various tests shown in the pull request closes https://github.com/official-stockfish/Stockfish/pull/3663 No functional change
201 lines
7.9 KiB
C++
201 lines
7.9 KiB
C++
/*
|
|
Stockfish, a UCI chess playing engine derived from Glaurung 2.1
|
|
Copyright (C) 2004-2021 The Stockfish developers (see AUTHORS file)
|
|
|
|
Stockfish is free software: you can redistribute it and/or modify
|
|
it under the terms of the GNU General Public License as published by
|
|
the Free Software Foundation, either version 3 of the License, or
|
|
(at your option) any later version.
|
|
|
|
Stockfish is distributed in the hope that it will be useful,
|
|
but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
GNU General Public License for more details.
|
|
|
|
You should have received a copy of the GNU General Public License
|
|
along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
// Definition of layer ClippedReLU of NNUE evaluation function
|
|
|
|
#ifndef NNUE_LAYERS_CLIPPED_RELU_H_INCLUDED
|
|
#define NNUE_LAYERS_CLIPPED_RELU_H_INCLUDED
|
|
|
|
#include "../nnue_common.h"
|
|
|
|
namespace Stockfish::Eval::NNUE::Layers {
|
|
|
|
// Clipped ReLU
|
|
template <typename PreviousLayer>
|
|
class ClippedReLU {
|
|
public:
|
|
// Input/output type
|
|
using InputType = typename PreviousLayer::OutputType;
|
|
using OutputType = std::uint8_t;
|
|
static_assert(std::is_same<InputType, std::int32_t>::value, "");
|
|
|
|
// Number of input/output dimensions
|
|
static constexpr IndexType InputDimensions = PreviousLayer::OutputDimensions;
|
|
static constexpr IndexType OutputDimensions = InputDimensions;
|
|
static constexpr IndexType PaddedOutputDimensions =
|
|
ceil_to_multiple<IndexType>(OutputDimensions, 32);
|
|
|
|
// Size of forward propagation buffer used in this layer
|
|
static constexpr std::size_t SelfBufferSize =
|
|
ceil_to_multiple(OutputDimensions * sizeof(OutputType), CacheLineSize);
|
|
|
|
// Size of the forward propagation buffer used from the input layer to this layer
|
|
static constexpr std::size_t BufferSize =
|
|
PreviousLayer::BufferSize + SelfBufferSize;
|
|
|
|
// Hash value embedded in the evaluation file
|
|
static constexpr std::uint32_t get_hash_value() {
|
|
std::uint32_t hashValue = 0x538D24C7u;
|
|
hashValue += PreviousLayer::get_hash_value();
|
|
return hashValue;
|
|
}
|
|
|
|
// Read network parameters
|
|
bool read_parameters(std::istream& stream) {
|
|
return previousLayer.read_parameters(stream);
|
|
}
|
|
|
|
// Write network parameters
|
|
bool write_parameters(std::ostream& stream) const {
|
|
return previousLayer.write_parameters(stream);
|
|
}
|
|
|
|
// Forward propagation
|
|
const OutputType* propagate(
|
|
const TransformedFeatureType* transformedFeatures, char* buffer) const {
|
|
const auto input = previousLayer.propagate(
|
|
transformedFeatures, buffer + SelfBufferSize);
|
|
const auto output = reinterpret_cast<OutputType*>(buffer);
|
|
|
|
#if defined(USE_AVX2)
|
|
if constexpr (InputDimensions % SimdWidth == 0) {
|
|
constexpr IndexType NumChunks = InputDimensions / SimdWidth;
|
|
const __m256i Zero = _mm256_setzero_si256();
|
|
const __m256i Offsets = _mm256_set_epi32(7, 3, 6, 2, 5, 1, 4, 0);
|
|
const auto in = reinterpret_cast<const __m256i*>(input);
|
|
const auto out = reinterpret_cast<__m256i*>(output);
|
|
for (IndexType i = 0; i < NumChunks; ++i) {
|
|
const __m256i words0 = _mm256_srai_epi16(_mm256_packs_epi32(
|
|
_mm256_load_si256(&in[i * 4 + 0]),
|
|
_mm256_load_si256(&in[i * 4 + 1])), WeightScaleBits);
|
|
const __m256i words1 = _mm256_srai_epi16(_mm256_packs_epi32(
|
|
_mm256_load_si256(&in[i * 4 + 2]),
|
|
_mm256_load_si256(&in[i * 4 + 3])), WeightScaleBits);
|
|
_mm256_store_si256(&out[i], _mm256_permutevar8x32_epi32(_mm256_max_epi8(
|
|
_mm256_packs_epi16(words0, words1), Zero), Offsets));
|
|
}
|
|
} else {
|
|
constexpr IndexType NumChunks = InputDimensions / (SimdWidth / 2);
|
|
const __m128i Zero = _mm_setzero_si128();
|
|
const auto in = reinterpret_cast<const __m128i*>(input);
|
|
const auto out = reinterpret_cast<__m128i*>(output);
|
|
for (IndexType i = 0; i < NumChunks; ++i) {
|
|
const __m128i words0 = _mm_srai_epi16(_mm_packs_epi32(
|
|
_mm_load_si128(&in[i * 4 + 0]),
|
|
_mm_load_si128(&in[i * 4 + 1])), WeightScaleBits);
|
|
const __m128i words1 = _mm_srai_epi16(_mm_packs_epi32(
|
|
_mm_load_si128(&in[i * 4 + 2]),
|
|
_mm_load_si128(&in[i * 4 + 3])), WeightScaleBits);
|
|
const __m128i packedbytes = _mm_packs_epi16(words0, words1);
|
|
_mm_store_si128(&out[i], _mm_max_epi8(packedbytes, Zero));
|
|
}
|
|
}
|
|
constexpr IndexType Start =
|
|
InputDimensions % SimdWidth == 0
|
|
? InputDimensions / SimdWidth * SimdWidth
|
|
: InputDimensions / (SimdWidth / 2) * (SimdWidth / 2);
|
|
|
|
#elif defined(USE_SSE2)
|
|
constexpr IndexType NumChunks = InputDimensions / SimdWidth;
|
|
|
|
#ifdef USE_SSE41
|
|
const __m128i Zero = _mm_setzero_si128();
|
|
#else
|
|
const __m128i k0x80s = _mm_set1_epi8(-128);
|
|
#endif
|
|
|
|
const auto in = reinterpret_cast<const __m128i*>(input);
|
|
const auto out = reinterpret_cast<__m128i*>(output);
|
|
for (IndexType i = 0; i < NumChunks; ++i) {
|
|
const __m128i words0 = _mm_srai_epi16(_mm_packs_epi32(
|
|
_mm_load_si128(&in[i * 4 + 0]),
|
|
_mm_load_si128(&in[i * 4 + 1])), WeightScaleBits);
|
|
const __m128i words1 = _mm_srai_epi16(_mm_packs_epi32(
|
|
_mm_load_si128(&in[i * 4 + 2]),
|
|
_mm_load_si128(&in[i * 4 + 3])), WeightScaleBits);
|
|
const __m128i packedbytes = _mm_packs_epi16(words0, words1);
|
|
_mm_store_si128(&out[i],
|
|
|
|
#ifdef USE_SSE41
|
|
_mm_max_epi8(packedbytes, Zero)
|
|
#else
|
|
_mm_subs_epi8(_mm_adds_epi8(packedbytes, k0x80s), k0x80s)
|
|
#endif
|
|
|
|
);
|
|
}
|
|
constexpr IndexType Start = NumChunks * SimdWidth;
|
|
|
|
#elif defined(USE_MMX)
|
|
constexpr IndexType NumChunks = InputDimensions / SimdWidth;
|
|
const __m64 k0x80s = _mm_set1_pi8(-128);
|
|
const auto in = reinterpret_cast<const __m64*>(input);
|
|
const auto out = reinterpret_cast<__m64*>(output);
|
|
for (IndexType i = 0; i < NumChunks; ++i) {
|
|
const __m64 words0 = _mm_srai_pi16(
|
|
_mm_packs_pi32(in[i * 4 + 0], in[i * 4 + 1]),
|
|
WeightScaleBits);
|
|
const __m64 words1 = _mm_srai_pi16(
|
|
_mm_packs_pi32(in[i * 4 + 2], in[i * 4 + 3]),
|
|
WeightScaleBits);
|
|
const __m64 packedbytes = _mm_packs_pi16(words0, words1);
|
|
out[i] = _mm_subs_pi8(_mm_adds_pi8(packedbytes, k0x80s), k0x80s);
|
|
}
|
|
_mm_empty();
|
|
constexpr IndexType Start = NumChunks * SimdWidth;
|
|
|
|
#elif defined(USE_NEON)
|
|
constexpr IndexType NumChunks = InputDimensions / (SimdWidth / 2);
|
|
const int8x8_t Zero = {0};
|
|
const auto in = reinterpret_cast<const int32x4_t*>(input);
|
|
const auto out = reinterpret_cast<int8x8_t*>(output);
|
|
for (IndexType i = 0; i < NumChunks; ++i) {
|
|
int16x8_t shifted;
|
|
const auto pack = reinterpret_cast<int16x4_t*>(&shifted);
|
|
pack[0] = vqshrn_n_s32(in[i * 2 + 0], WeightScaleBits);
|
|
pack[1] = vqshrn_n_s32(in[i * 2 + 1], WeightScaleBits);
|
|
out[i] = vmax_s8(vqmovn_s16(shifted), Zero);
|
|
}
|
|
constexpr IndexType Start = NumChunks * (SimdWidth / 2);
|
|
#else
|
|
constexpr IndexType Start = 0;
|
|
#endif
|
|
|
|
for (IndexType i = Start; i < InputDimensions; ++i) {
|
|
output[i] = static_cast<OutputType>(
|
|
std::max(0, std::min(127, input[i] >> WeightScaleBits)));
|
|
}
|
|
|
|
// Affine transform layers expect that there is at least
|
|
// ceil_to_multiple(OutputDimensions, 32) initialized values.
|
|
// We cannot do this in the affine transform because it requires
|
|
// preallocating space here.
|
|
for (IndexType i = OutputDimensions; i < PaddedOutputDimensions; ++i) {
|
|
output[i] = 0;
|
|
}
|
|
|
|
return output;
|
|
}
|
|
|
|
private:
|
|
PreviousLayer previousLayer;
|
|
};
|
|
|
|
} // namespace Stockfish::Eval::NNUE::Layers
|
|
|
|
#endif // NNUE_LAYERS_CLIPPED_RELU_H_INCLUDED
|